-->
— Event scheduler. Source code: Lib/sched.py. The sched module defines a class which implements a general purpose event scheduler: The scheduler class defines a generic interface to scheduling events. It needs two functions to actually deal with the “outside world” — timefunc should be callable without arguments, and return a. Question or problem about Python programming: I am trying to properly understand and implement two concurrently running Task objects using Python 3’s relatively new asyncio module. In a nutshell, asyncio seems designed to handle asynchronous processes and concurrent Task execution over an event loop. It promotes the use of await (applied in async functions) as.
Azure Pipelines Car disassembly 3d hack apk.
Use this task to run a Python script.
Arguments
Argument | Description |
---|---|
scriptSource Type | (Required) Target script type: File path or Inline |
scriptPath Script Path | (Required when scriptSource filePath ) Path of the script to execute. Must be a fully qualified path or relative to $(System.DefaultWorkingDirectory). |
script Script | (Required when scriptSource inline ) The Python script to run |
arguments Arguments | (Optional) A string containing arguments passed to the script. They'll be available through sys.argv as if you passed them on the command line. |
pythonInterpreter Python interpreter | (Optional) Absolute path to the Python interpreter to use. If not specified, the task assumes a Python interpreter is available on the PATH and simply attempts to run the python command. |
workingDirectory Working directory | (Optional) |
failOnStderr Fail on standard error | (Optional) If true, this task will fail if any text is written to stderr . |
Remarks
By default, this task will invoke python
from the system path.Run Use Python Version to put the version you want in the system path.
Open source
This task is open source on GitHub. Feedback and contributions are welcome.
Source code:Lib/sched.py
The sched
module defines a class which implements a general purpose eventscheduler:
sched.
scheduler
(timefunc=time.monotonic, delayfunc=time.sleep)¶The scheduler
class defines a generic interface to scheduling events.It needs two functions to actually deal with the “outside world” — timefuncshould be callable without arguments, and return a number (the “time”, in anyunits whatsoever). The delayfunc function should be callable with oneargument, compatible with the output of timefunc, and should delay that manytime units. delayfunc will also be called with the argument 0
after eachevent is run to allow other threads an opportunity to run in multi-threadedapplications.
Changed in version 3.3: timefunc and delayfunc parameters are optional.
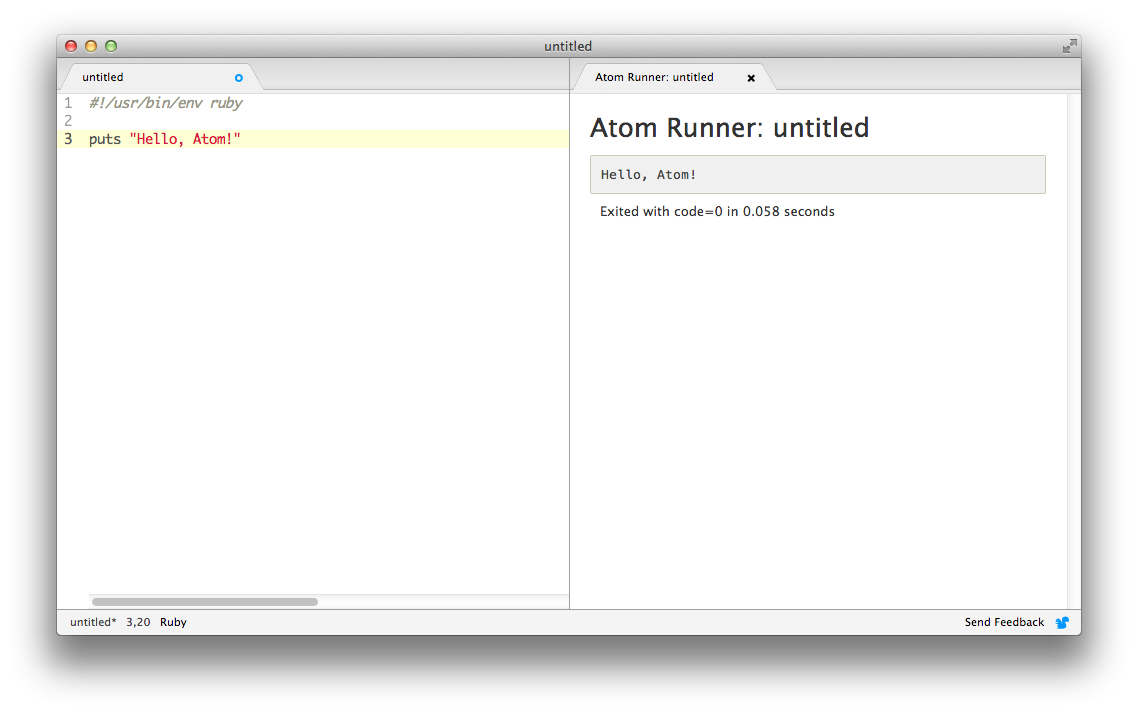
Changed in version 3.3: scheduler
class can be safely used in multi-threadedenvironments.
Example:
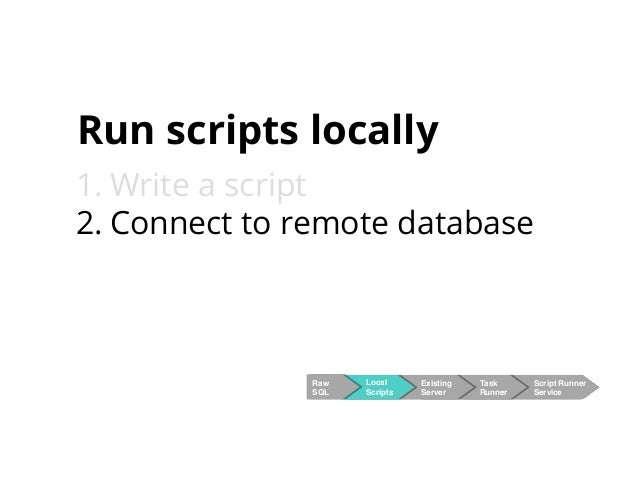
Scheduler Objects¶
scheduler
instances have the following methods and attributes:
scheduler.
enterabs
(time, priority, action, argument=(), kwargs={})¶Schedule a new event. The time argument should be a numeric type compatiblewith the return value of the timefunc function passed to the constructor.Events scheduled for the same time will be executed in the order of theirpriority. A lower number represents a higher priority.
Executing the event means executing action(*argument,**kwargs)
.argument is a sequence holding the positional arguments for action.kwargs is a dictionary holding the keyword arguments for action.
Return value is an event which may be used for later cancellation of the event(see cancel()
).

Changed in version 3.3: argument parameter is optional.
Changed in version 3.3: kwargs parameter was added.
scheduler.
enter
(delay, priority, action, argument=(), kwargs={})¶Schedule an event for delay more time units. Frutiger font family free mac. Other than the relative time, theother arguments, the effect and the return value are the same as those forenterabs()
.
Python Task Runner Free
Changed in version 3.3: argument parameter is optional.
Changed in version 3.3: kwargs parameter was added.
scheduler.
cancel
(event)¶Remove the event from the queue. If event is not an event currently in thequeue, this method will raise a ValueError
.
scheduler.
empty
()¶Return True
if the event queue is empty.
scheduler.
run
(blocking=True)¶Run all scheduled events. This method will wait (using the delayfunc()
function passed to the constructor) for the next event, then execute it and soon until there are no more scheduled events.
If blocking is false executes the scheduled events due to expire soonest(if any) and then return the deadline of the next scheduled call in thescheduler (if any).
Either action or delayfunc can raise an exception. In either case, thescheduler will maintain a consistent state and propagate the exception. If anexception is raised by action, the event will not be attempted in future callsto run()
.
Python Task Runner Download
If a sequence of events takes longer to run than the time available before thenext event, the scheduler will simply fall behind. No events will be dropped;the calling code is responsible for canceling events which are no longerpertinent.
Changed in version 3.3: blocking parameter was added.
scheduler.
queue
¶Close All Running Tasks
Read-only attribute returning a list of upcoming events in the order theywill be run. Each event is shown as a named tuple with thefollowing fields: time, priority, action, argument, kwargs.